AI Assistant Fills in Business Logic Code Based on Generated Code Templates
Tips
Requires sponge v1.13.0+ version
Overview
With the popularization of AI technology, AI-assisted programming (such as Cursor, Windsurf, Trae, etc.) has become an important part of developers' work. Using AI-assisted programming tools indeed improves development efficiency, especially when generating code for single simple functions. However, these tools still have significant limitations when dealing with complex business scenarios, mainly reflected in their insufficient understanding of frameworks, the fact that generated code often requires manual adjustments, and high integration costs. The specific pain points are as follows:
- Demand decomposition relies on manual effort: AI is currently unable to independently decompose complex requirements and still requires developers to clearly break down tasks and provide detailed guidance.
- Insufficient context understanding: When dealing with large projects or complex business logic, it is difficult for AI to accurately capture the context, leading to generated code deviating from expectations.
- Maintainability risks: Generated code may have inconsistent styles or not match the existing project structure, increasing the burden of future maintenance.
- High Prompt sensitivity: The output quality heavily depends on the precision of input instructions; vague Prompts can easily lead to inefficient results.
The essence of these problems is that current AI is still in the code imitation phase and lacks project-level global modeling capabilities. Although future technological development is expected to gradually solve these issues, more practical solutions are still needed at present.
Why Does sponge Integrate an AI Assistant?
Most AI tools on the market lack deep integration with specific Go development frameworks. Here, "deep integration" means that AI tools are not just surface-level code assistants but can deeply understand the framework's structure, conventions, and best practices, providing code generation and modification capabilities highly consistent with the framework. They can generate code according to the directory structure and file organization conventions agreed upon by the framework. This deep integration significantly reduces developer context switching and manual integration work, allowing AI-generated code to be "plug-and-play".
The sponge framework is deeply integrated with the built-in AI assistant to address some of the pain points encountered when using AI tools for project development, helping developers implement business logic more easily and quickly. Its features are as follows:
- The sponge development framework is deeply integrated with mainstream AI models (DeepSeek, ChatGPT, Gemini);
- Generates code according to the directory structure and file organization conventions agreed upon by the sponge framework, and the generated code style is consistent with the sponge framework code style;
- The AI assistant supports concurrent code generation for multiple tasks, improving code generation efficiency.
- Supports one-click code merging to minimize manual integration of Go code.
Applicable scenarios: Use the built-in AI assistant to quickly generate business logic code when a service created with sponge lacks business logic code.
Comparison of code generation capabilities of mainstream large language models (from the internet, tested April 2025, for reference only):
Topic | o3-mini-high | Claude 3.7 | DeepSeek-V3-0324 | Gemini 2.5 Pro |
---|---|---|---|---|
Medium Algorithm Problem | ✅ | ❌ | ❌ | ✅ |
Hard Algorithm Problem | ✅ | ❌ | ❌ | ✅ |
Super Mario | ✅ | ✅ | ✅ | ✅ |
3D Maze | ❌ | ❌ | ❌ | ✅ |
Generate Code
Preparation
Before use, please obtain the API Key for the corresponding platform. You can choose one or more platforms.
Conditions to Trigger sponge's Built-in AI Assistant for Code Generation
- Define a function in Go code
- Add detailed function comments (serving as AI prompts)
- Add the
panic("implement me")
marker within the function body
Example:
// Describe the specific functionality the function needs to implement
func MyFunc() {
panic("implement me")
}
Using the AI Assistant for Code Generation in a sponge Project
In services created with sponge based on protobuf, the generated code automatically meets the conditions to trigger the AI assistant for code generation, eliminating the need to manually create Go functions. The specific steps are as follows:
First, add detailed comments to the
.proto
methods in therpc
file. These comments will serve as AI prompts and are recommended to include business rules and technical points.Then execute the
make proto
command to generate corresponding.go
files in theinternal/handler
orinternal/service
directories. The code in these files will contain Go functions that meet the conditions for AI assistant code generation.proto file example:
syntax = "proto3"; package api.user.v1; service User { // User login verification // - Supports account/password and SMS login // - Password needs encrypted verification // - SMS validity needs verification rpc Login(LoginRequest) returns (LoginReply) {} }
Use the built-in AI assistant in sponge to generate code:
- Open the sponge UI interface;
- Navigate to the left-hand menu [AI Assistant Generate Code] → [Generate Go Code];
- Fill in the following parameters (hover over the question mark
?
next to the parameter to see parameter descriptions):- Large Language Type: e.g.,
deepseek
- Model: e.g.,
deepseek-chat
- API Key: e.g.,
sk-xxxxxx
- Working Directory: e.g.,
/home/gopher/project/user
- Large Language Type: e.g.,
- Click the Generate Code button, as shown in the figure below:
Generate Code Example Equivalent Command
sponge assistant generate --type=deepseek --model=deepseek-chat --api-key=sk-xxxxxx --dir=/home/gopher/project/user
Tips
The Go code generated by the AI assistant is saved in markdown files with the suffix
.xxx.md
in the same directory as the.go
file, where xxx is the name of the large language type (e.g., deepseek, chatgpt, gemini).In the code generation feature page, you can specify the generation scope in two ways:
Working Directory Mode:
- The AI Assistant will process all
.go
files within the specified directory, identify all eligible functions, and generate the implementation code. - Note: If the directory contains a large number of eligible functions, the generation process may take a longer time. The specific duration depends on the processing performance of the AI large language model.
- The AI Assistant will process all
Go File Mode (Recommended):
- In this mode, the AI Assistant will only process a specified single
.go
file, identify all eligible functions within it, and generate the implementation code. - This more targeted approach is better suited to the needs of real-world development scenarios.
- In this mode, the AI Assistant will only process a specified single
If you are not satisfied with the generated result, you can adjust the function comment description, or switch models and generate again, and compare the code quality of different models.
Merge Code
The logic code generated by the AI assistant is initially saved in .xxx.md
files. If confirmed to be correct, you can use the automatic merging function provided by sponge to quickly merge it into the corresponding .go
file. The specific steps are as follows:
- Open the sponge UI interface;
- Navigate to the left-hand menu [AI Assistant Generate Code] → [Merge Go Code];
- Fill in the following parameters (hover over the question mark
?
next to the parameter to see parameter descriptions):- Large Language Type: e.g.,
deepseek
. If code has been generated by multiple large language types, select the one with the most satisfactory code. - Working Directory: e.g.,
/home/gopher/project/user
- Clean AI Code: Default is
true
. Whether to automatically delete.xxx.md
files after merging.
- Large Language Type: e.g.,
- Click the Merge Code button to complete the code integration, as shown in the figure below:
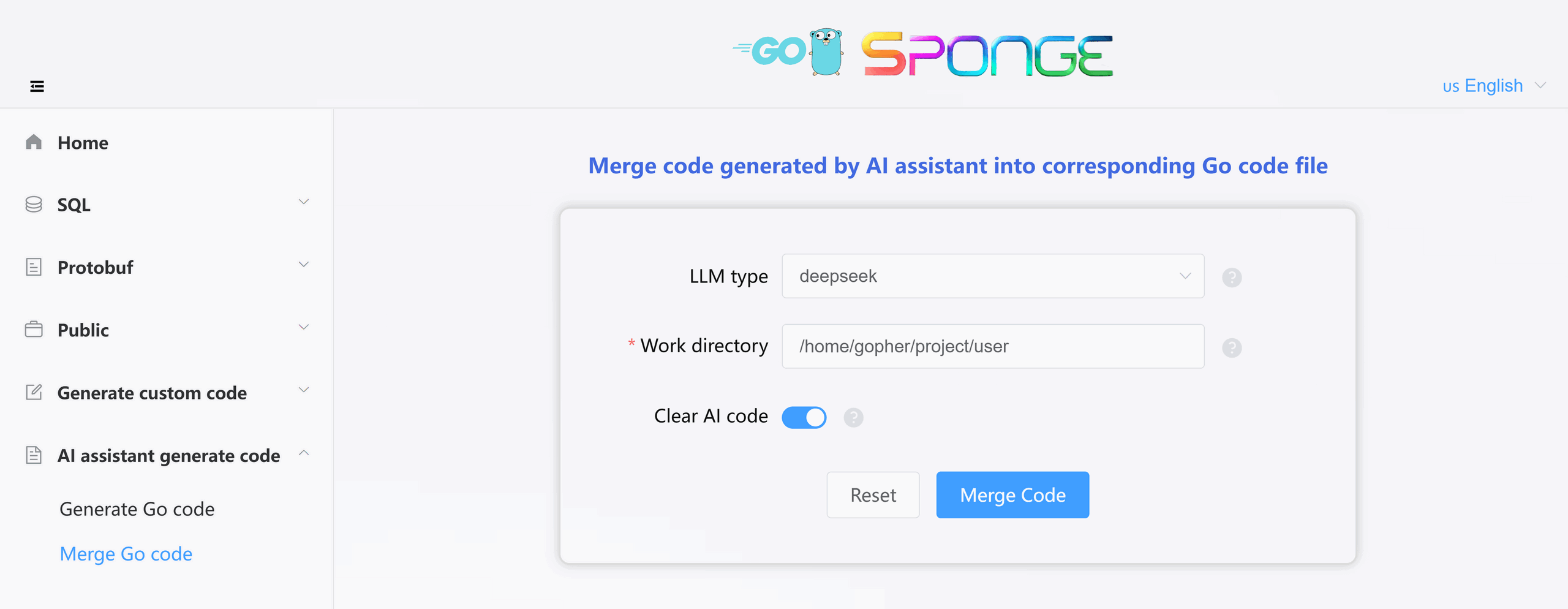
Equivalent Command
sponge assistant merge --type=deepseek --dir=/home/gopher/project/user --is-clean=true
Tips
Before merging code, the original .go
file will be automatically backed up to prevent code loss due to modifications. After successful merging, the path where the backup code is stored will be printed. If you need to restore the code, you can manually recover the .go
file.
Important
If you merge code using different models multiple times, the result of the later merge will overwrite the content of the previous merge.
Clean AI Generated Files
To batch clean up all .xxx.md
AI generated files, you can execute the following command:
sponge assistant clean --dir=/path/to/directory