Generating Code Based on JSON + Custom Templates
Tips
Requires sponge v1.11.0+
Overview
Generating code based on custom templates and fields is a flexible and efficient approach. Since template code and fields are entirely defined by the user, this method is suitable for generating commonly used, repeatable code of any type, such as boilerplate code, microservice code, configuration files, deployment scripts, etc.
Creating Custom Fields
Custom fields are the input parameters for template code generation. First, you need to define the field names and their corresponding values in a JSON file, for example, fields.json
:
{
"ServerName": "web",
"PackageName": "main",
"HandlerName": "helloworld",
"Port": 8080
}
Each field will correspond to a placeholder in the template code.
Creating Custom Template Code
Template code is the core of code generation, implemented using Go's text/template
library. Therefore, it's recommended to first familiarize yourself with its basic syntax rules. The syntax rules are simple and can be learned in a few minutes. Click to view the chapter: Go text/template Basic Syntax Rules.
When creating template files, you can use fixed file names (like main.go
) or variable file names (like {{.ServerName}}.go.tmpl
). Below is an example of template file content:
package {{.PackageName}}
import (
"net/http"
)
// {{.HandlerName}} handles {{.HandlerName}} requests
func {{.HandlerName}}(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Hello, world!"))
}
func main() {
http.HandleFunc("/{{.HandlerName}}", {{.HandlerName}})
if err := http.ListenAndServe(":{{.Port}}", nil); err != nil {
panic(err)
}
}
Store template files in a directory (e.g., template
), which can contain multiple template files and subdirectories.
Generating Code
Execute the command sponge run
in the terminal to enter the code generation UI interface. Here are the specific steps:
- Go to the left menu [Generate Custom Code] → [JSON];
- Fill in the following parameters (hover over the question mark
?
next to the parameter to view the parameter description):- Custom Template Directory: e.g.,
D:\template\web
- Custom Fields: e.g.,
fields.json
- Custom Template Directory: e.g.,
- Click the Download Code button to generate and download the code, as shown in the figure below:
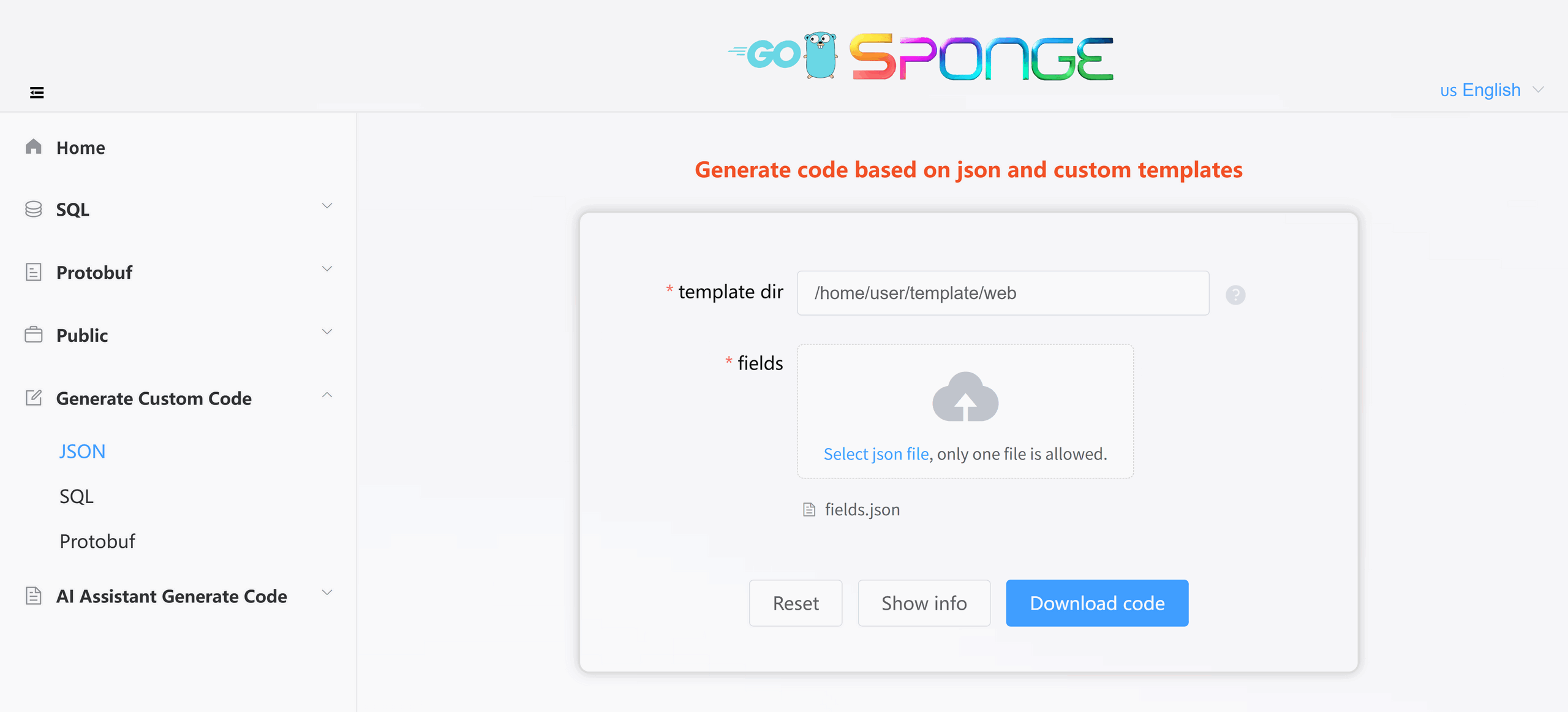
Equivalent Command
sponge template field --tpl-dir=D:\template\web --fields=fields.json
Tips
Click the button View Template Info
to view the field information. This field information corresponds to the template's placeholders, making it more convenient to write template code.